Passing an argument to a slot. Ask Question Asked 8 years, 8 months ago. How to use this if my context parameter targets a to a class which has no acess to the actions? So eitherway. How to pass a value with a clicked signal from a Qt PushButton?
I have an old codebase I started writing using the Qt 3.x framework—a little while before Qt4 was released. It’s still alive! I still work on it, keeping up-to-date with Qt and C++ as much as possible, and I still ship the product. Over the years I have moved the codebase along through Qt4 to Qt5, and on to compilers supporting C++11. One of the things I’ve sometimes found a little excessive is declaring slots for things that are super short and won’t be reused.
Here’s a simplified example from my older code that changes the title when a project is “dirty” (needs to be saved):
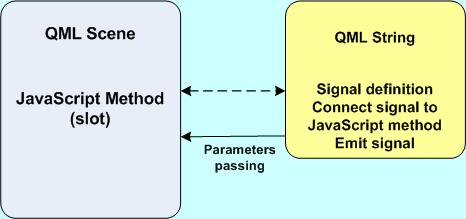
2 | &myMainWindow::slotProjectDirtyChanged ); |
Already an improvement!
Qt Signal Slot Arguments
What are these lambda things anyways?

A lambda is essentially a fancy name for an anonymous function. These are typically short and can be useful for things like this slot mechanism where the code may not be re-usable, or for callbacks.
The C++11 specification for a lambda function looks like this:
[capture](parameters) -> return_type { function_body }
Looks simple, but when you dig into the details, they can be rather complicated.
The capture
part defines how variables in the current scope are captured for the function_body to use. Put another way, it defines what variables are made available to the lambda function body from the current scope.
The one we’ll use here is [=]
which says to capture all variables from the local scope by value. You can also capture nothing ([]
), capture all variables in the scope by reference ([&]
*note), capture just the members of the class ([this]
), or capture individual variables ([foo, &bar]
) – or some combination.
It also takes optional parameters
which is how we’ll pass signal parameters to the lambda (see below).
return_type
and function_body
are standard C++.
If we apply this to our example, we get something like this:
2 4 | connect(mNavView,&myNavView::signalNavBoxChange, [=] ( const QRectF &inRect ) { const QRectF cAdjusted( inRect.topLeft() / mRatio, inRect.bottomRight() / mRatio ); mView->slotMoveView(cAdjusted); |
You’ll note that I am also using mRatio and mView from the enclosing class. Because we captured using [=]
, the body of the lambda function has access to variables in the enclosing scope.
Because I’m only using the members of this
, I could have captured using [this]
instead: